Reachable Set
ReachableSetInterface
ReachableSetInterface
class
- class commonroad_reach.data_structure.reach.reach_interface.ReachableSetInterface(config)[source]
Interface for reachable set computation.
- Parameters
config (Configuration) –
- property step_start
- property step_end
- property drivable_area
- property reachable_set
- reset(config)[source]
Resets configuration of the interface.
- Parameters
config (Configuration) –
- compute_reachable_sets(step_start=0, step_end=0)[source]
Computes reachable sets between the given start and end steps.
- Parameters
step_start (int) –
step_end (int) –
- extract_driving_corridors(to_goal_region=False, shape_terminal=None, is_cartesian_shape=True, corridor_lon=None, list_p_lon=None)[source]
Extracts driving corridors within the reachable sets.
- Parameters
to_goal_region (
bool
) – whether the corridors should intersect with the goal regionshape_terminal (
Optional
[Shape
]) – a user-specified shape representing the terminal stateis_cartesian_shape (
bool
) – whether the shape is given in Cartesian coordinate systemcorridor_lon (
Optional
[DrivingCorridor
]) – a longitudinal driving corridorlist_p_lon (
Optional
[List
[float
]]) – a list of longitudinal positions
- Return type
List
[DrivingCorridor
]- Returns
a list of driving corridors
ReachNode
ReachNode
class
- class commonroad_reach.data_structure.reach.reach_node.ReachNode(polygon_lon, polygon_lat, step=- 1)[source]
Node within a reachability graph, also used in reachable set computations.
Note
Each node is a Cartesian product of longitudinal and lateral polygons.
Curvilinear coordinate system: polygon_lon is a polygon in the longitudinal p-v domain, and polygon_lat is a polygon in the lateral p-v domain.
Cartesian coordinate system: polygons are in the x-v and y-v domains, respectively.
- Parameters
polygon_lon (ReachPolygon) –
polygon_lat (ReachPolygon) –
step (int) –
- cnt_id = 0
- property polygon_lon: ReachPolygon
Polygon in the longitudinal direction. See note of
ReachNode
.- Return type
- property polygon_lat: ReachPolygon
Polygon in the lateral direction. See note of
ReachNode
.- Return type
- property p_lon_min
Minimum position in the longitudinal direction.
- property p_lon_max
Maximum position in the longitudinal direction.
- property v_lon_min
Minimum velocity in the longitudinal direction.
- property v_lon_max
Maximum velocity in the longitudinal direction.
- property p_lat_min
Minimum position in the lateral direction.
- property p_lat_max
Maximum position in the lateral direction.
- property v_lat_min
Minimum velocity in the lateral direction.
- property v_lat_max
Maximum velocity in the lateral direction.
- property p_x_min
Minimum x-position in the Cartesian coordinate system.
- property p_x_max
Maximum x-position in the Cartesian coordinate system.
- property v_x_min
Minimum x-velocity in the Cartesian coordinate system.
- property v_x_max
Maximum x-velocity in the Cartesian coordinate system.
- property p_y_min
Minimum y-position in the Cartesian coordinate system.
- property p_y_max
Maximum y-position in the Cartesian coordinate system.
- property v_y_min
Minimum y-velocity in the Cartesian coordinate system.
- property v_y_max
Maximum y-velocity in the Cartesian coordinate system.
- update_position_rectangle()[source]
Updates the position rectangle based on the latest position attributes.
- translate(p_lon_off=0.0, v_lon_off=0.0, p_lat_off=0.0, v_lat_off=0.0)[source]
Returns a copy translated by input offsets.
- Parameters
p_lon_off (float) –
v_lon_off (float) –
p_lat_off (float) –
v_lat_off (float) –
- intersect_in_position_domain(p_lon_min, p_lat_min, p_lon_max, p_lat_max)[source]
Perform intersection in the position domain.
- Parameters
p_lon_min (float) –
p_lat_min (float) –
p_lon_max (float) –
p_lat_max (float) –
ReachNodeMultiGeneration
class
- class commonroad_reach.data_structure.reach.reach_node.ReachNodeMultiGeneration(polygon_lon, polygon_lat, step=- 1)[source]
Node within a reachability graph, also used in reachable set computations.
In addition to
ReachNode
, this class holds lists reach nodes across generations.- Parameters
step (int) –
- add_grandparent_node(node_grandparent)[source]
- Return type
bool
- Parameters
node_grandparent (ReachNodeMultiGeneration) –
- remove_grandparent_node(node_grandparent)[source]
- Return type
bool
- Parameters
node_grandparent (ReachNodeMultiGeneration) –
- add_grandchild_node(node_grandchild)[source]
- Return type
bool
- Parameters
node_grandchild (ReachNodeMultiGeneration) –
- position_rectangle: Optional[ReachPolygon]
- remove_grandchild_node(node_grandchild)[source]
- Return type
bool
- Parameters
node_grandchild (ReachNodeMultiGeneration) –
ReachPolygon
ReachPolygon
class
- class commonroad_reach.data_structure.reach.reach_polygon.ReachPolygon(list_vertices, fix_vertices=True)[source]
Polygon class that constitutes reach nodes and position rectangles.
Note
When used to represent a reach node, it is defined in the position-velocity domain, and can be used to represent a polygon in either the longitudinal or the lateral direction.
When used to represent a position rectangle, it is defined in the longitudinal/lateral position domain.
- Parameters
list_vertices (list) –
- property p_min
Minimum position in the position-velocity domain.
- property p_max
Maximum position in the position-velocity domain.
- property v_min
Minimum velocity in the position-velocity domain.
- property v_max
Maximum velocity in the position-velocity domain.
- property p_lon_min
Minimum longitudinal position in the position domain.
- property p_lon_max
Maximum longitudinal position in the position domain.
- property p_lon_center
Center longitudinal position in the position domain.
- property p_lat_min
Minimum lateral position in the position domain.
- property p_lat_max
Maximum lateral position in the position domain.
- property p_lat_center
Center lateral position in the position domain.
- property diagonal_squared
Square length of the diagonal of the position domain.
- property vertices: List[Tuple[ndarray, ndarray]]
Returns the list of vertices of the polygon.
- Return type
List
[Tuple
[ndarray
,ndarray
]]
- clone(convexify)[source]
Returns a cloned (and convexified) polygon.
- Return type
- Parameters
convexify (bool) –
- intersect_halfspace(a, b, c)[source]
Returns the intersection of the polygon and the halfspace specified in the form of ax + by <= c.
- Return type
Optional
[ReachPolygon
]- Parameters
a (float) –
b (float) –
c (float) –
- classmethod from_polygon(polygon)[source]
Returns a polygon constructed from the vertices of the given polygon.
- Return type
Optional
[ReachPolygon
]- Parameters
polygon (Polygon) –
- static from_rectangle_vertices(p_lon_min, p_lat_min, p_lon_max, p_lat_max)[source]
Returns a polygon given the vertices of a rectangle.
- Return type
- Parameters
p_lon_min (float) –
p_lat_min (float) –
p_lon_max (float) –
p_lat_max (float) –
- static get_vertices(polygon)[source]
Returns the list of vertices of the polygon.
- Return type
List
[Tuple
[ndarray
,ndarray
]]- Parameters
polygon (Union[Polygon, ReachPolygon]) –
- static construct_halfspace_polygon(a, b, c, bounds_polygon)[source]
Returns a polygon representing the halfspace.
Note
General case: First compute two arbitrary vertices that are far away from the x boundary, then compute the slope of the vector that is perpendicular to the vector connecting these two points to look for remaining two vertices required for the polygon construction.
- Return type
Polygon
- Parameters
a (float) –
b (float) –
c (float) –
bounds_polygon (Tuple[float, float, float, float]) –
ReachableSet
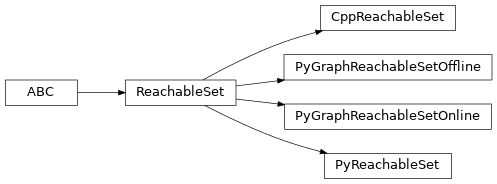
ReachableSet
class
- class commonroad_reach.data_structure.reach.reach_set.ReachableSet(config)[source]
Abstract superclass for reachable sets.
- Parameters
config (Configuration) –
- property drivable_area
- property reachable_set
- abstract compute(step_start, step_end)[source]
Computes reachable set between the specified start and end steps.
- Parameters
step_start (int) –
step_end (int) –
- classmethod instantiate(config)[source]
Instantiates a reachable set class based on the given configuration.
- Parameters
config (Configuration) –
CppReachableSet
CppReachableSet
class
- class commonroad_reach.data_structure.reach.reach_set_cpp.CppReachableSet(config)[source]
Reachable set computation with C++ backend.
- Parameters
config (Configuration) –
PyReachableSet
PyReachableSet
class
- class commonroad_reach.data_structure.reach.reach_set_py.PyReachableSet(config)[source]
Reachable set computation with Python backend.
- Parameters
config (Configuration) –
PyGraphReachableSetOffline
PyGraphReachableSetOffline
class
- class commonroad_reach.data_structure.reach.reach_set_py_graph_offline.PyGraphReachableSetOffline(config)[source]
Offline step in the graph-based reachable set computation with Python backend.
- Parameters
config (Configuration) –
- property path_offline_file
PyGraphReachableSetOnline
PyGraphReachableSetOnline
class
- class commonroad_reach.data_structure.reach.reach_set_py_graph_online.PyGraphReachableSetOnline(config)[source]
Online step in the graph-based reachable set computation with Python backend.
- Parameters
config (Configuration) –
- property max_evaluated_step: int
- Return type
int
- drivable_area_at_step(step)[source]
- Return type
List
[ReachPolygon
]- Parameters
step (int) –
- reachable_set_at_step(step)[source]
- Return type
List
[ReachNodeMultiGeneration
]- Parameters
step (int) –
- time_step(time_index)[source]
Converts relative time index (initial time_index = 0) to time_step (initial step = step_start)
- Return type
int
- Parameters
time_index (int) –
- reachset_translation(step)[source]
Translation of initial state at the given step.
- Return type
ndarray
- Parameters
step (int) –
- initialize_new_scenario(scenario=None, planning_problem=None)[source]
Resets online computation for evaluation of new scenario and/or planning problem; thus, avoid time for parsing pickle file again.
- Parameters
scenario (Optional[Scenario]) – new scenario (keep old scenario if None)
planning_problem ([Optional]) – new planning_problem (keep old planning_problem if None)
DrivingCorridor
ConnectedComponent
class
DrivingCorridor
class
- class commonroad_reach.data_structure.reach.driving_corridor.DrivingCorridor[source]
Class representing a sequence of
ConnectedComponent
.- property step_final
- add_connected_component(cc)[source]
- Parameters
cc (ConnectedComponent) –
DrivingCorridorExtractor
DrivingCorridorExtractor
class
- class commonroad_reach.data_structure.reach.driving_corridor_extractor.DrivingCorridorExtractor(reachable_sets, config)[source]
Class to extract driving corridors from reachable sets and drivable areas.
- Parameters
reachable_sets (Dict[int, List[Union[ReachNode, ReachNode]]]) –
config (Configuration) –
- property steps
- extract(to_goal_region=False, shape_terminal=None, is_cartesian_shape=True, corridor_lon=None, list_p_lon=None)[source]
Extracts driving corridors within the reachable sets.
If a longitudinal DC and a list of positions are given, lateral DCs are extracted. Otherwise, proceed to longitudinal DC extraction. Optionally, one can specify whether the longitudinal DC should reach the goal region of the planning problem or a user-given terminal states represented by a shape.
- Parameters
to_goal_region (
bool
) – whether a driving corridor should end in the goal region of the planning problemshape_terminal (
Optional
[Shape
]) – terminal positions represented by a CR Shape objectis_cartesian_shape (
bool
) – flag indicating whether the shape is described in Cartesian coordinate systemcorridor_lon (
Optional
[DrivingCorridor
]) – a longitudinal driving corridorlist_p_lon (
Optional
[List
[float
]]) – a list of positions in the longitudinal direction
- Return type
List
[DrivingCorridor
]- Returns
a list of extracted driving corridors